{"value":"NOTE: This post assumes the reader has some familiarity with the [AWS Cloud Development Kit (CDK)](https://aws.amazon.com/cdk/). To get started with the [AWS CDK](https://aws.amazon.com/cn/cdk/?trk=cndc-detail), please visit the [AWS CDK Developer Guide](https://docs.aws.amazon.com/cdk/v2/guide/home.html) and follow the [AWS CDK for .NET workshop](https://cdkworkshop.com/40-dotnet.html).\n\nThe [AWS CDK](https://aws.amazon.com/cn/cdk/?trk=cndc-detail) is an an open-source Infrastructure-as-Code (IaC) framework that allows developers to model and provision cloud application resources using the language of their choice. .NET developers can write their IaC project in C# using [AWS CDK](https://aws.amazon.com/cn/cdk/?trk=cndc-detail) for .NET and add it to their Solution as a Console Application. This allows developers to leverage their existing skills and familiarity with Visual Studio and the .NET Ecosystem to build cloud infrastructure along side the rest of their application.\n\nWe often get questions from customers asking how they can debug their CDK projects directly from Visual Studio. Even though CDK does not support this functionality at this time, with a little judicious use of [System.Diagnostics.Debugger](https://docs.microsoft.com/en-us/dotnet/api/system.diagnostics.debugger?view=net-5.0), we’ll be debugging in no time! In this post, we will show you how to do this step-by-step.\n\n#### **Debugger.Launch()**\n\nTo start debugging, add a call to [Debugger.Launch()](https://docs.microsoft.com/en-us/dotnet/api/system.diagnostics.debugger.launch?view=net-5.0#System_Diagnostics_Debugger_Launch) inside your CDK project’s Program ```Main``` method.\n\nC#\n\n```\\nnamespace Cdk\\n{\\n class Program\\n {\\n public static void Main(string[] args)\\n {\\n // note: will launch debugger every time. See below for full example.\\n Debugger.Launch();\\n\\n var app = new App();\\n new TVCampaignStack(app, \\"TVCampaignStack\\", new StackProps());\\n app.Synth();\\n }\\n }\\n }\\n```\nWhen invoked, the debugger will pause execution and pop a dialog box inviting the user to attach Visual Studio.\n\n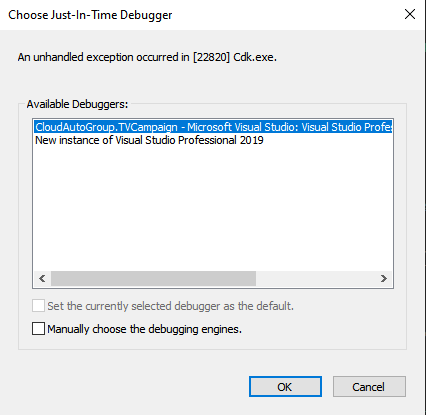\n\nOnce attached, you can debug your Infrastructure directly from Visual Studio!\n\n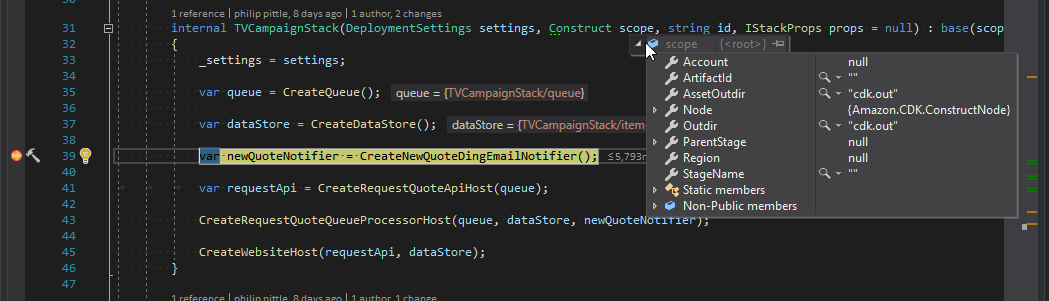\n\n#### **Config Driven**\n\nWhile it’s very handy to be able to launch and attach a Visual Studio debugger, we don’t want this to happen every time, especially if deploying from a CI/CD system or other automated tooling.\n\nThere is a number of ways to inject configuration into an [AWS CDK](https://aws.amazon.com/cn/cdk/?trk=cndc-detail) for .NET project. The most straight forward way is to pass [Context](Context) in from the command line. We can modify our example from above like this:\n\nC#\n\n```\\npublic static void Main(string[] args)\\n{\\n var app = new App();\\n \\n bool.TryParse(app.Node.TryGetContext(\\"debug\\")?.ToString(), out var debug);\\n\\n if (debug)\\n Debugger.Launch();\\n\\n new TVCampaignStack(app, \\"TVCampaignStack\\", new StackProps());\\n app.Synth();\\n}\\n```\n\nNow we can control when the debugger will launch via the command line:\n\nPowerShell\n\n```\\n# Launches the Debugger\\nPS C:\\\\Example> cdk deploy -c debug=true\\n\\n# Does not launch debugger\\nPS C:\\\\Example> cdk deploy\\n```\n#### **Summary**\n\nWith a well placed call to ```Debugger.Launch()``` inside our Program’s ```Main``` method, debugging your C# CDK Infrastructure inside of Visual Studio becomes easy!\n\n#### **Further Reading**\n\n[AWS CDK](https://aws.amazon.com/cn/cdk/?trk=cndc-detail) for .NET Workshop: [https://cdkworkshop.com/40-dotnet.html](https://cdkworkshop.com/40-dotnet.html)\nIaC in C# with [AWS CDK](https://aws.amazon.com/cn/cdk/?trk=cndc-detail): [https://www.youtube.com/watch?v=5aBf0W0_FDY](https://www.youtube.com/watch?v=5aBf0W0_FDY)\nCDK Context: [https://docs.aws.amazon.com/cdk/v2/guide/context.html](https://docs.aws.amazon.com/cdk/v2/guide/context.html)\nAlternative Configuration Example: [https://github.com/ppittle/cdk-talk-2021/blob/main/CloudAutoGroup/cdk/Program.cs#L41](https://github.com/ppittle/cdk-talk-2021/blob/main/CloudAutoGroup/cdk/Program.cs#L41)\n\n\n\n","render":"<p>NOTE: This post assumes the reader has some familiarity with the <a href=\\"https://aws.amazon.com/cdk/\\" target=\\"_blank\\">AWS Cloud Development Kit (CDK)</a>. To get started with the [AWS CDK](https://aws.amazon.com/cn/cdk/?trk=cndc-detail), please visit the <a href=\\"https://docs.aws.amazon.com/cdk/v2/guide/home.html\\" target=\\"_blank\\">AWS CDK Developer Guide</a> and follow the <a href=\\"https://cdkworkshop.com/40-dotnet.html\\" target=\\"_blank\\">AWS CDK for .NET workshop</a>.</p>\\n<p>The AWS CDK is an an open-source Infrastructure-as-Code (IaC) framework that allows developers to model and provision cloud application resources using the language of their choice. .NET developers can write their IaC project in C# using AWS CDK for .NET and add it to their Solution as a Console Application. This allows developers to leverage their existing skills and familiarity with Visual Studio and the .NET Ecosystem to build cloud infrastructure along side the rest of their application.</p>\n<p>We often get questions from customers asking how they can debug their CDK projects directly from Visual Studio. Even though CDK does not support this functionality at this time, with a little judicious use of <a href=\\"https://docs.microsoft.com/en-us/dotnet/api/system.diagnostics.debugger?view=net-5.0\\" target=\\"_blank\\">System.Diagnostics.Debugger</a>, we’ll be debugging in no time! In this post, we will show you how to do this step-by-step.</p>\\n<h4><a id=\\"DebuggerLaunch_6\\"></a><strong>Debugger.Launch()</strong></h4>\\n<p>To start debugging, add a call to <a href=\\"https://docs.microsoft.com/en-us/dotnet/api/system.diagnostics.debugger.launch?view=net-5.0#System_Diagnostics_Debugger_Launch\\" target=\\"_blank\\">Debugger.Launch()</a> inside your CDK project’s Program <code>Main</code> method.</p>\\n<p>C#</p>\n<pre><code class=\\"lang-\\">namespace Cdk\\n{\\n class Program\\n {\\n public static void Main(string[] args)\\n {\\n // note: will launch debugger every time. See below for full example.\\n Debugger.Launch();\\n\\n var app = new App();\\n new TVCampaignStack(app, "TVCampaignStack", new StackProps());\\n app.Synth();\\n }\\n }\\n }\\n</code></pre>\\n<p>When invoked, the debugger will pause execution and pop a dialog box inviting the user to attach Visual Studio.</p>\n<p><img src=\\"https://dev-media.amazoncloud.cn/c5ecac68144f43a1bf79576fbce07669_image.png\\" alt=\\"image.png\\" /></p>\n<p>Once attached, you can debug your Infrastructure directly from Visual Studio!</p>\n<p><img src=\\"https://dev-media.amazoncloud.cn/521ce6e2b1784d11b4d74149c8116811_image.png\\" alt=\\"image.png\\" /></p>\n<h4><a id=\\"Config_Driven_37\\"></a><strong>Config Driven</strong></h4>\\n<p>While it’s very handy to be able to launch and attach a Visual Studio debugger, we don’t want this to happen every time, especially if deploying from a CI/CD system or other automated tooling.</p>\n<p>There is a number of ways to inject configuration into an AWS CDK for .NET project. The most straight forward way is to pass <a href=\\"Context\\" target=\\"_blank\\">Context</a> in from the command line. We can modify our example from above like this:</p>\\n<p>C#</p>\n<pre><code class=\\"lang-\\">public static void Main(string[] args)\\n{\\n var app = new App();\\n \\n bool.TryParse(app.Node.TryGetContext("debug")?.ToString(), out var debug);\\n\\n if (debug)\\n Debugger.Launch();\\n\\n new TVCampaignStack(app, "TVCampaignStack", new StackProps());\\n app.Synth();\\n}\\n</code></pre>\\n<p>Now we can control when the debugger will launch via the command line:</p>\n<p>PowerShell</p>\n<pre><code class=\\"lang-\\"># Launches the Debugger\\nPS C:\\\\Example> cdk deploy -c debug=true\\n\\n# Does not launch debugger\\nPS C:\\\\Example> cdk deploy\\n</code></pre>\\n<h4><a id=\\"Summary_71\\"></a><strong>Summary</strong></h4>\\n<p>With a well placed call to <code>Debugger.Launch()</code> inside our Program’s <code>Main</code> method, debugging your C# CDK Infrastructure inside of Visual Studio becomes easy!</p>\\n<h4><a id=\\"Further_Reading_75\\"></a><strong>Further Reading</strong></h4>\\n<p>AWS CDK for .NET Workshop: <a href=\\"https://cdkworkshop.com/40-dotnet.html\\" target=\\"_blank\\">https://cdkworkshop.com/40-dotnet.html</a><br />\\nIaC in C# with AWS CDK: <a href=\\"https://www.youtube.com/watch?v=5aBf0W0_FDY\\" target=\\"_blank\\">https://www.youtube.com/watch?v=5aBf0W0_FDY</a><br />\\nCDK Context: <a href=\\"https://docs.aws.amazon.com/cdk/v2/guide/context.html\\" target=\\"_blank\\">https://docs.aws.amazon.com/cdk/v2/guide/context.html</a><br />\\nAlternative Configuration Example: <a href=\\"https://github.com/ppittle/cdk-talk-2021/blob/main/CloudAutoGroup/cdk/Program.cs#L41\\" target=\\"_blank\\">https://github.com/ppittle/cdk-talk-2021/blob/main/CloudAutoGroup/cdk/Program.cs#L41</a></p>\n"}