{"value":"Quarkus offers Java developers the capability of building native images based on [GraalVM](https://www.graalvm.org/). A native image is a binary that includes everything: your code, libraries, and a smaller virtual machine (VM). This approach improves the startup time of your [Amazon Web Services Lambda](https://aws.amazon.com/es/lambda/) functions, because it is optimized for container-based environments. These use cloud native and serverless architectures with a container-first philosophy.\n\nIn this blog post, you learn how to integrate the [Quarkus framework](https://quarkus.io/) with [Amazon Web Services Lambda functions, using the Amazon Web Services Serverless Application Model (Amazon Web Services SAM)](https://aws.amazon.com/serverless/sam/).\n\n#### **Reduce infrastructure costs and improve latency**\nWhen you develop applications with Quarkus and GraalVM with native images, the bootstrap file generated requires more time to compile, but it has a faster runtime. GraalVM is a JIT compiler that generates optimized native machine code that provides different garbage collector implementations, and uses less memory and CPU. This is achieved with a battery of advanced compiler optimizations and aggressive and sophisticated inlining techniques. By using Quarkus, you can also reduce your infrastructure costs because you need less resources.\n\nWith Quarkus and Amazon Web Services SAM features, you can improve the latency performance of your Java-based Amazon Web Services Lambda functions by reducing the cold-start time. A cold-start is the initialization time that a Lambda function takes before running the actual code. After the function is initialized for the first time, future requests will reuse the same execution environment without incurring the cold-start time, leading to improved performance.\n\n#### **Overview of solution**\nFigure 1 shows the Amazon Web Services components and workflow of our solution.\n\n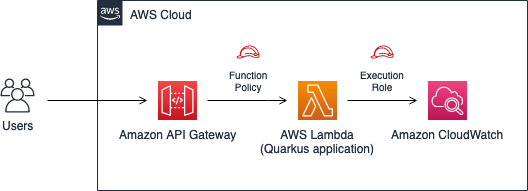\n\nFigure 1. Architecture diagram for Quarkus (Amazon Web Services Lambda) application\n\nWith Amazon Web Services SAM, you can easily integrate external frameworks by using custom runtimes and configuring properties in the template file and the Makefile.\n\n#### **Prerequisites**\nFor this walkthrough, you should have the following prerequisites:\n\n- Software components: Java 11 JDK (like [Amazon Corretto](https://aws.amazon.com/corretto/)), Maven, and the latest [Amazon Web Services SAM CLI](https://docs.aws.amazon.com/serverless-application-model/latest/developerguide/serverless-sam-cli-install.html).\n- Admin permissions in the following services: Amazon Web Services Lambda, [Amazon CloudWatch](https://aws.amazon.com/cloudwatch/), [Amazon API Gateway](https://aws.amazon.com/api-gateway/), and [Amazon Web Services Identity and Access Management](https://aws.amazon.com/iam/). You should consider least privilege principle in your own use case as a best practice.\n\n#### **Creating a Java-based Amazon Web Services Lambda function**\nAmazon Web Services SAM provides default templates to accelerate the development of new functions. Create a Java-based function by following these steps:\n\nRun the following command in your terminal:\n```\\nsam init -a x86_64 -r java11 -p Zip -d maven -n java11-mvn-default\\n```\n\nThese parameters select a x86 architecture, java11 as Java runtime LTS version, Zip as a build artifact, and Maven as the package and dependency tool. It also defines the project name.\n\nChoose the first option to use a template for your base code:\n1. Amazon Web Services Quick Start Templates\nFinally, with the previous selection you have different templates to choose from to create the base structure of your function. In our case, select the first one, which creates an Amazon Web Services Lambda function calling an external HTTPS endpoint. This will get the IP address and return it with a “Hello World” response to the user in JSON:\n\n2. Hello World Example\nThe output will yield the following, shown in Figure 2:\n\n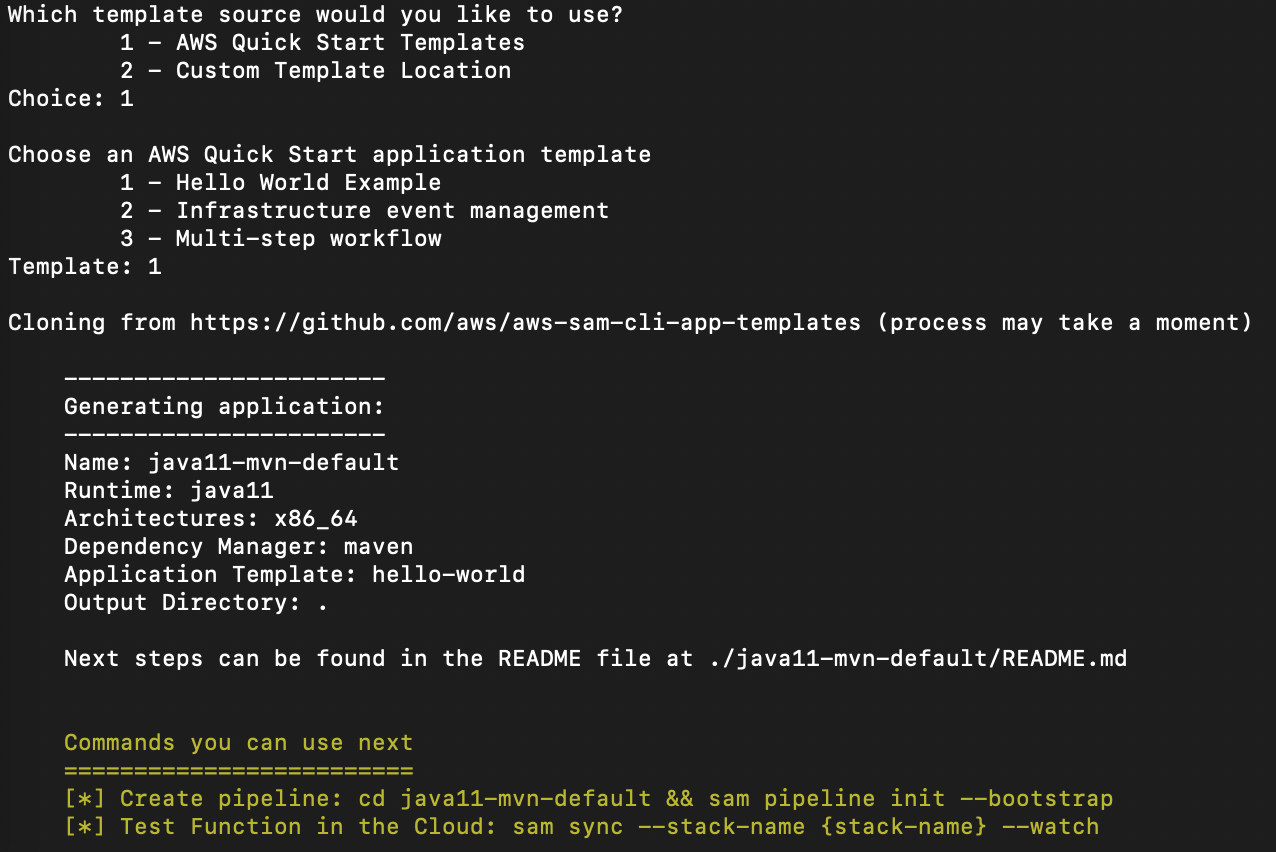\n\nFigure 2. Amazon Web Services SAM configuration input data\n\n#### **Integrating Quarkus framework\n**Using Amazon Web Services SAM, you can easily integrate non-Amazon Web Services [custom runtimes](https://docs.aws.amazon.com/serverless-application-model/latest/developerguide/building-custom-runtimes.html) in your Amazon Web Services Lambda functions. With this feature, you can integrate the Quarkus framework. Follow the next four steps:\n\n**1. Create a Makefile file**\n\nCreate a “Makefile” file in the “HelloWorldFunction” directory with this code:\n\n```\\nbuild-HelloWorldFunction:\\n mvn clean package -Pnative -Dquarkus.native.container-build=true -Dquarkus.native.builder-image=quay.io/quarkus/ubi-quarkus-mandrel:21.3-java11\\n @ unzip ./target/function.zip -d \$(ARTIFACTS_DIR)\\n```\n\nWith this snippet, you are configuring Amazon Web Services SAM to build the bootstrap runtime using Maven instructions for Amazon Web Services SAM.\n\nUsing Quarkus, you can build a Linux executable without having to install GraalVM with the next option:\n\n```\\n-Dquarkus.native.container-build=true\\n```\n\nFor more information, you can visit the official site and learn more about [building a native image](https://quarkus.io/guides/building-native-image).\n\n**2. Configure Maven dependencies**\n\nAs a Maven project, include the necessary dependencies. Change the pom.xml file in the “HelloWorldFunction” directory to remove the default libraries:\n\n```\\n<dependencies>\\n <dependency>\\n <groupId>com.amazonaws</groupId>\\n <artifactId>aws-lambda-java-core</artifactId>\\n <version>1.2.1</version>\\n </dependency>\\n <dependency>\\n <groupId>com.amazonaws</groupId>\\n <artifactId>aws-lambda-java-events</artifactId>\\n <version>3.6.0</version>\\n </dependency>\\n</dependencies>\\n```\n\nAdd the Quarkus libraries, profile, and plugins in the right pom.xml section as shown in the following XML configuration. At the current time, the latest version of Quarkus is 2.7.1.Final. We highly recommend using the latest versions of the libraries and plugins:\n\n```\\n<dependencies>\\n <dependency>\\n <groupId>io.quarkus</groupId>\\n <artifactId>quarkus-amazon-lambda</artifactId>\\n <version>2.7.1.Final</version>\\n </dependency>\\n <dependency>\\n <groupId>io.quarkus</groupId>\\n <artifactId>quarkus-arc</artifactId>\\n <version>2.7.1.Final</version>\\n </dependency>\\n <dependency>\\n <groupId>junit</groupId>\\n <artifactId>junit</artifactId>\\n <version>4.13.1</version>\\n <scope>test</scope>\\n </dependency>\\n</dependencies>\\n\\n<build>\\n <finalName>function</finalName>\\n <plugins>\\n <plugin>\\n <groupId>io.quarkus</groupId>\\n <artifactId>quarkus-maven-plugin</artifactId>\\n <version>2.7.1.Final</version>\\n <extensions>true</extensions>\\n <executions>\\n <execution>\\n <goals>\\n <goal>build</goal>\\n <goal>generate-code</goal>\\n <goal>generate-code-tests</goal>\\n </goals>\\n </execution>\\n </executions>\\n </plugin>\\n </plugins>\\n</build>\\n\\n<profiles>\\n <profile>\\n <id>native</id>\\n <activation>\\n <property>\\n <name>native</name>\\n </property>\\n </activation>\\n <properties>\\n <quarkus.package.type>native</quarkus.package.type>\\n </properties>\\n </profile>\\n</profiles>\\n```\n\n**3. Configure the template.yaml to use the previous Makefile**\n\nTo configure the Amazon Web Services SAM template to use your own Makefile configuration using Quarkus and Maven instructions correctly, edit the template.yaml file to add the following properties:\n```\\nResources:\\n HelloWorldFunction:\\n Metadata:\\n BuildMethod: makefile\\n Properties:\\n Runtime: provided\\n```\n\n**4. Add a new properties file to enable SSL configuration**\n\nFinally, create an application.properties file in the directory: ../HelloWorldFunction/src/main/resources/ with the following property:\n```\\nquarkus.ssl.native=true\\n```\nThis property is needed because the sample function uses a secure connection to [https://checkip.amazonaws.com](https://checkip.amazonaws.com/). It will get the response body in the sample you selected previously.\n\nNow you can build and deploy your first Quarkus function with the following Amazon Web Services SAM commands:\n```\\nsam build\\n```\nThis will create the Zip artifact using the Maven tool and will build the native image to deploy on Amazon Web Services Lambda based on your previous Makefile configuration. Finally, run the following Amazon Web Services SAM command to deploy your function:\n\n```\\nsam deploy -–guided\\n```\nThe first time you deploy an Amazon Web Services SAM application, you can customize some configurations or parameters like the Stack name, the Amazon Web Services Region, and more (see Figure 3). You can also accept the default one. For more information about Amazon Web Services SAM deploy options, read the [Amazon Web Services SAM documentation](https://docs.aws.amazon.com/serverless-application-model/latest/developerguide/sam-cli-command-reference-sam-deploy.html).\n\n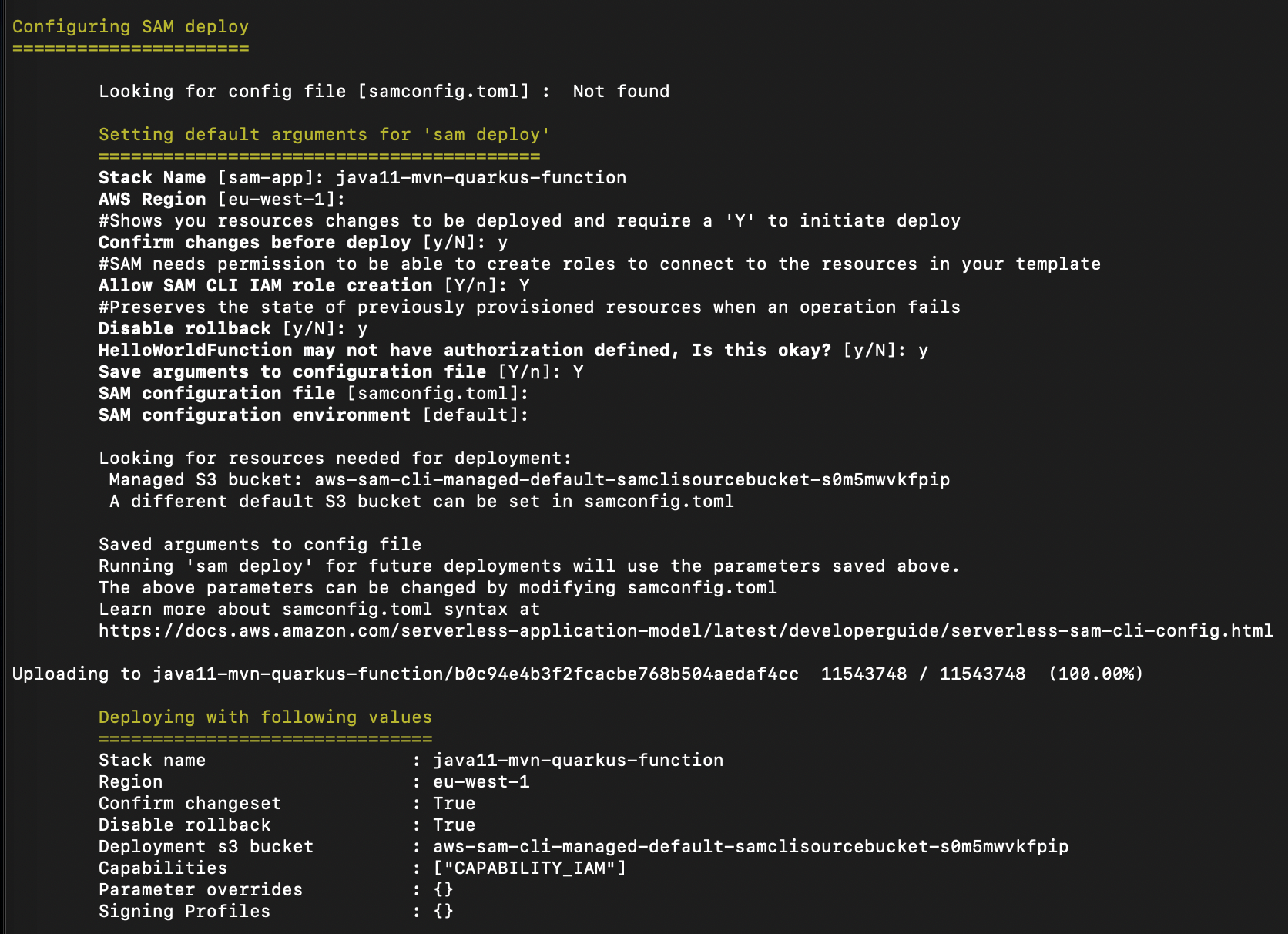\n\nFigure 3. Lambda deployment configuration input data\n\nThis sample configuration enables you to configure the necessary IAM permissions to deploy the Amazon Web Services SAM resources for this sample. After completing the task, you can see the Amazon Web Services CloudFormation Stack and resources created by Amazon Web Services SAM.\n\nYou have now created and deployed an HTTPS API Gateway endpoint with a Quarkus application on Amazon Web Services Lambda that you can test.\n\n#### **Testing your Quarkus function**\n\nFinally, test your Quarkus function in the [Amazon Web Services Management Console](https://aws.amazon.com/console/) by selecting the new function in the Amazon Web Services Lambda functions list. Use the test feature included in the console, as shown in Figure 4:\n\n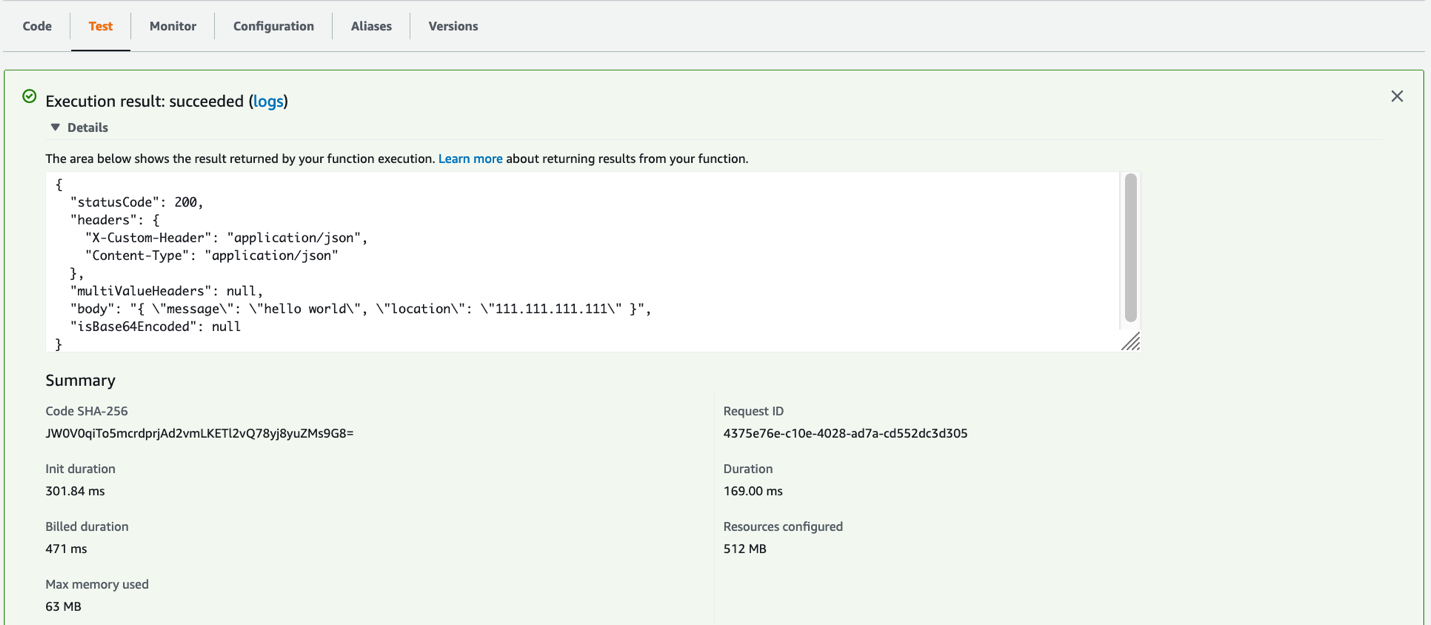\n\nFigure 4. Lambda execution test example\n\nYou will get a response to your Lambda request and a summary. This includes information like duration, or resources needed in your new Quarkus function. For more information about testing applications on Amazon Web Services SAM, you can read [Testing and debugging serverless applications](https://docs.aws.amazon.com/serverless-application-model/latest/developerguide/serverless-test-and-debug.html). You can also visit the official site to read more information using [Amazon Web Services SAM with Quarkus](https://quarkus.io/guides/amazon-lambda#testing-with-the-sam-cli).\n\n#### **Cleaning up**\nTo avoid incurring future charges, delete the resources created in your Amazon Web Services Lambda stack. You can delete resources with the following command:\n```\\nsam delete\\n```\n#### **Conclusion**\nIn this post, we demonstrated how to integrate Java frameworks like Quarkus on Amazon Web Services Lambda using custom runtimes with Amazon Web Services SAM. This enables you to configure custom build configurations or your preferred frameworks. These tools improve the developer experience, standardizing the tool used to develop serverless applications with future requirements, showing a strong flexibility for developers.\n\nThe Quarkus native image generated and applied in the Amazon Web Services Lambda function reduces the heavy Java footprint. You can use your Java skills to develop serverless applications without having to change the programming language. This is a great advantage when cold-starts or compute resources are important for business or technical requirements.\n\n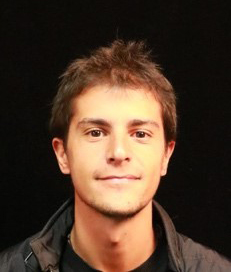\n\n**Joan Bonilla**\nJoan Bonilla is a Solutions Architect in Iberia for the public sector. He has been developing software for 10 years and is a passionate about serverless and cloud-native architectures.\n\n\n\n\n\n\n\n\n","render":"<p>Quarkus offers Java developers the capability of building native images based on <a href=\\"https://www.graalvm.org/\\" target=\\"_blank\\">GraalVM</a>. A native image is a binary that includes everything: your code, libraries, and a smaller virtual machine (VM). This approach improves the startup time of your <a href=\\"https://aws.amazon.com/es/lambda/\\" target=\\"_blank\\">Amazon Web Services Lambda</a> functions, because it is optimized for container-based environments. These use cloud native and serverless architectures with a container-first philosophy.</p>\\n<p>In this blog post, you learn how to integrate the <a href=\\"https://quarkus.io/\\" target=\\"_blank\\">Quarkus framework</a> with <a href=\\"https://aws.amazon.com/serverless/sam/\\" target=\\"_blank\\">Amazon Web Services Lambda functions, using the Amazon Web Services Serverless Application Model (Amazon Web Services SAM)</a>.</p>\\n<h4><a id=\\"Reduce_infrastructure_costs_and_improve_latency_4\\"></a><strong>Reduce infrastructure costs and improve latency</strong></h4>\\n<p>When you develop applications with Quarkus and GraalVM with native images, the bootstrap file generated requires more time to compile, but it has a faster runtime. GraalVM is a JIT compiler that generates optimized native machine code that provides different garbage collector implementations, and uses less memory and CPU. This is achieved with a battery of advanced compiler optimizations and aggressive and sophisticated inlining techniques. By using Quarkus, you can also reduce your infrastructure costs because you need less resources.</p>\n<p>With Quarkus and Amazon Web Services SAM features, you can improve the latency performance of your Java-based Amazon Web Services Lambda functions by reducing the cold-start time. A cold-start is the initialization time that a Lambda function takes before running the actual code. After the function is initialized for the first time, future requests will reuse the same execution environment without incurring the cold-start time, leading to improved performance.</p>\n<h4><a id=\\"Overview_of_solution_9\\"></a><strong>Overview of solution</strong></h4>\\n<p>Figure 1 shows the Amazon Web Services components and workflow of our solution.</p>\n<p><img src=\\"https://dev-media.amazoncloud.cn/1a605cdb29424b35a659621aa897dee6_image.png\\" alt=\\"image.png\\" /></p>\n<p>Figure 1. Architecture diagram for Quarkus (Amazon Web Services Lambda) application</p>\n<p>With Amazon Web Services SAM, you can easily integrate external frameworks by using custom runtimes and configuring properties in the template file and the Makefile.</p>\n<h4><a id=\\"Prerequisites_18\\"></a><strong>Prerequisites</strong></h4>\\n<p>For this walkthrough, you should have the following prerequisites:</p>\n<ul>\\n<li>Software components: Java 11 JDK (like <a href=\\"https://aws.amazon.com/corretto/\\" target=\\"_blank\\">Amazon Corretto</a>), Maven, and the latest <a href=\\"https://docs.aws.amazon.com/serverless-application-model/latest/developerguide/serverless-sam-cli-install.html\\" target=\\"_blank\\">Amazon Web Services SAM CLI</a>.</li>\\n<li>Admin permissions in the following services: Amazon Web Services Lambda, <a href=\\"https://aws.amazon.com/cloudwatch/\\" target=\\"_blank\\">Amazon CloudWatch</a>, <a href=\\"https://aws.amazon.com/api-gateway/\\" target=\\"_blank\\">Amazon API Gateway</a>, and <a href=\\"https://aws.amazon.com/iam/\\" target=\\"_blank\\">Amazon Web Services Identity and Access Management</a>. You should consider least privilege principle in your own use case as a best practice.</li>\\n</ul>\n<h4><a id=\\"Creating_a_Javabased_Amazon_Web_Services_Lambda_function_24\\"></a><strong>Creating a Java-based Amazon Web Services Lambda function</strong></h4>\\n<p>Amazon Web Services SAM provides default templates to accelerate the development of new functions. Create a Java-based function by following these steps:</p>\n<p>Run the following command in your terminal:</p>\n<pre><code class=\\"lang-\\">sam init -a x86_64 -r java11 -p Zip -d maven -n java11-mvn-default\\n</code></pre>\\n<p>These parameters select a x86 architecture, java11 as Java runtime LTS version, Zip as a build artifact, and Maven as the package and dependency tool. It also defines the project name.</p>\n<p>Choose the first option to use a template for your base code:</p>\n<ol>\\n<li>\\n<p>Amazon Web Services Quick Start Templates<br />\\nFinally, with the previous selection you have different templates to choose from to create the base structure of your function. In our case, select the first one, which creates an Amazon Web Services Lambda function calling an external HTTPS endpoint. This will get the IP address and return it with a “Hello World” response to the user in JSON:</p>\n</li>\\n<li>\\n<p>Hello World Example<br />\\nThe output will yield the following, shown in Figure 2:</p>\n</li>\\n</ol>\n<p><img src=\\"https://dev-media.amazoncloud.cn/2e0397f494424e6580b2f55c5dd18764_image.png\\" alt=\\"image.png\\" /></p>\n<p>Figure 2. Amazon Web Services SAM configuration input data</p>\n<h4><a id=\\"Integrating_Quarkus_framework_45\\"></a>**Integrating Quarkus framework</h4>\\n<p>**Using Amazon Web Services SAM, you can easily integrate non-Amazon Web Services <a href=\\"https://docs.aws.amazon.com/serverless-application-model/latest/developerguide/building-custom-runtimes.html\\" target=\\"_blank\\">custom runtimes</a> in your Amazon Web Services Lambda functions. With this feature, you can integrate the Quarkus framework. Follow the next four steps:</p>\\n<p><strong>1. Create a Makefile file</strong></p>\\n<p>Create a “Makefile” file in the “HelloWorldFunction” directory with this code:</p>\n<pre><code class=\\"lang-\\">build-HelloWorldFunction:\\n mvn clean package -Pnative -Dquarkus.native.container-build=true -Dquarkus.native.builder-image=quay.io/quarkus/ubi-quarkus-mandrel:21.3-java11\\n @ unzip ./target/function.zip -d \$(ARTIFACTS_DIR)\\n</code></pre>\\n<p>With this snippet, you are configuring Amazon Web Services SAM to build the bootstrap runtime using Maven instructions for Amazon Web Services SAM.</p>\n<p>Using Quarkus, you can build a Linux executable without having to install GraalVM with the next option:</p>\n<pre><code class=\\"lang-\\">-Dquarkus.native.container-build=true\\n</code></pre>\\n<p>For more information, you can visit the official site and learn more about <a href=\\"https://quarkus.io/guides/building-native-image\\" target=\\"_blank\\">building a native image</a>.</p>\\n<p><strong>2. Configure Maven dependencies</strong></p>\\n<p>As a Maven project, include the necessary dependencies. Change the pom.xml file in the “HelloWorldFunction” directory to remove the default libraries:</p>\n<pre><code class=\\"lang-\\"><dependencies>\\n <dependency>\\n <groupId>com.amazonaws</groupId>\\n <artifactId>aws-lambda-java-core</artifactId>\\n <version>1.2.1</version>\\n </dependency>\\n <dependency>\\n <groupId>com.amazonaws</groupId>\\n <artifactId>aws-lambda-java-events</artifactId>\\n <version>3.6.0</version>\\n </dependency>\\n</dependencies>\\n</code></pre>\\n<p>Add the Quarkus libraries, profile, and plugins in the right pom.xml section as shown in the following XML configuration. At the current time, the latest version of Quarkus is 2.7.1.Final. We highly recommend using the latest versions of the libraries and plugins:</p>\n<pre><code class=\\"lang-\\"><dependencies>\\n <dependency>\\n <groupId>io.quarkus</groupId>\\n <artifactId>quarkus-amazon-lambda</artifactId>\\n <version>2.7.1.Final</version>\\n </dependency>\\n <dependency>\\n <groupId>io.quarkus</groupId>\\n <artifactId>quarkus-arc</artifactId>\\n <version>2.7.1.Final</version>\\n </dependency>\\n <dependency>\\n <groupId>junit</groupId>\\n <artifactId>junit</artifactId>\\n <version>4.13.1</version>\\n <scope>test</scope>\\n </dependency>\\n</dependencies>\\n\\n<build>\\n <finalName>function</finalName>\\n <plugins>\\n <plugin>\\n <groupId>io.quarkus</groupId>\\n <artifactId>quarkus-maven-plugin</artifactId>\\n <version>2.7.1.Final</version>\\n <extensions>true</extensions>\\n <executions>\\n <execution>\\n <goals>\\n <goal>build</goal>\\n <goal>generate-code</goal>\\n <goal>generate-code-tests</goal>\\n </goals>\\n </execution>\\n </executions>\\n </plugin>\\n </plugins>\\n</build>\\n\\n<profiles>\\n <profile>\\n <id>native</id>\\n <activation>\\n <property>\\n <name>native</name>\\n </property>\\n </activation>\\n <properties>\\n <quarkus.package.type>native</quarkus.package.type>\\n </properties>\\n </profile>\\n</profiles>\\n</code></pre>\\n<p><strong>3. Configure the template.yaml to use the previous Makefile</strong></p>\\n<p>To configure the Amazon Web Services SAM template to use your own Makefile configuration using Quarkus and Maven instructions correctly, edit the template.yaml file to add the following properties:</p>\n<pre><code class=\\"lang-\\">Resources:\\n HelloWorldFunction:\\n Metadata:\\n BuildMethod: makefile\\n Properties:\\n Runtime: provided\\n</code></pre>\\n<p><strong>4. Add a new properties file to enable SSL configuration</strong></p>\\n<p>Finally, create an application.properties file in the directory: …/HelloWorldFunction/src/main/resources/ with the following property:</p>\n<pre><code class=\\"lang-\\">quarkus.ssl.native=true\\n</code></pre>\\n<p>This property is needed because the sample function uses a secure connection to <a href=\\"https://checkip.amazonaws.com/\\" target=\\"_blank\\">https://checkip.amazonaws.com</a>. It will get the response body in the sample you selected previously.</p>\\n<p>Now you can build and deploy your first Quarkus function with the following Amazon Web Services SAM commands:</p>\n<pre><code class=\\"lang-\\">sam build\\n</code></pre>\\n<p>This will create the Zip artifact using the Maven tool and will build the native image to deploy on Amazon Web Services Lambda based on your previous Makefile configuration. Finally, run the following Amazon Web Services SAM command to deploy your function:</p>\n<pre><code class=\\"lang-\\">sam deploy -–guided\\n</code></pre>\\n<p>The first time you deploy an Amazon Web Services SAM application, you can customize some configurations or parameters like the Stack name, the Amazon Web Services Region, and more (see Figure 3). You can also accept the default one. For more information about Amazon Web Services SAM deploy options, read the <a href=\\"https://docs.aws.amazon.com/serverless-application-model/latest/developerguide/sam-cli-command-reference-sam-deploy.html\\" target=\\"_blank\\">Amazon Web Services SAM documentation</a>.</p>\\n<p><img src=\\"https://dev-media.amazoncloud.cn/aad3cf2f7f6f4b0f872806c8012743dc_image.png\\" alt=\\"image.png\\" /></p>\n<p>Figure 3. Lambda deployment configuration input data</p>\n<p>This sample configuration enables you to configure the necessary IAM permissions to deploy the Amazon Web Services SAM resources for this sample. After completing the task, you can see the Amazon Web Services CloudFormation Stack and resources created by Amazon Web Services SAM.</p>\n<p>You have now created and deployed an HTTPS API Gateway endpoint with a Quarkus application on Amazon Web Services Lambda that you can test.</p>\n<h4><a id=\\"Testing_your_Quarkus_function_184\\"></a><strong>Testing your Quarkus function</strong></h4>\\n<p>Finally, test your Quarkus function in the <a href=\\"https://aws.amazon.com/console/\\" target=\\"_blank\\">Amazon Web Services Management Console</a> by selecting the new function in the Amazon Web Services Lambda functions list. Use the test feature included in the console, as shown in Figure 4:</p>\\n<p><img src=\\"https://dev-media.amazoncloud.cn/9476b469ca9f45be9b0878ee8935a621_image.png\\" alt=\\"image.png\\" /></p>\n<p>Figure 4. Lambda execution test example</p>\n<p>You will get a response to your Lambda request and a summary. This includes information like duration, or resources needed in your new Quarkus function. For more information about testing applications on Amazon Web Services SAM, you can read <a href=\\"https://docs.aws.amazon.com/serverless-application-model/latest/developerguide/serverless-test-and-debug.html\\" target=\\"_blank\\">Testing and debugging serverless applications</a>. You can also visit the official site to read more information using <a href=\\"https://quarkus.io/guides/amazon-lambda#testing-with-the-sam-cli\\" target=\\"_blank\\">Amazon Web Services SAM with Quarkus</a>.</p>\\n<h4><a id=\\"Cleaning_up_194\\"></a><strong>Cleaning up</strong></h4>\\n<p>To avoid incurring future charges, delete the resources created in your Amazon Web Services Lambda stack. You can delete resources with the following command:</p>\n<pre><code class=\\"lang-\\">sam delete\\n</code></pre>\\n<h4><a id=\\"Conclusion_199\\"></a><strong>Conclusion</strong></h4>\\n<p>In this post, we demonstrated how to integrate Java frameworks like Quarkus on Amazon Web Services Lambda using custom runtimes with Amazon Web Services SAM. This enables you to configure custom build configurations or your preferred frameworks. These tools improve the developer experience, standardizing the tool used to develop serverless applications with future requirements, showing a strong flexibility for developers.</p>\n<p>The Quarkus native image generated and applied in the Amazon Web Services Lambda function reduces the heavy Java footprint. You can use your Java skills to develop serverless applications without having to change the programming language. This is a great advantage when cold-starts or compute resources are important for business or technical requirements.</p>\n<p><img src=\\"https://dev-media.amazoncloud.cn/2a71a93ba5444472aa058703474d7ac7_image.png\\" alt=\\"image.png\\" /></p>\n<p><strong>Joan Bonilla</strong><br />\\nJoan Bonilla is a Solutions Architect in Iberia for the public sector. He has been developing software for 10 years and is a passionate about serverless and cloud-native architectures.</p>\n"}